Diameter Lua Service
Introduction
The DiameterLuaService is a service for initiating Lua scripts running within the LogicApp.
The DiameterLuaService receives messages from one or more instances of the DiameterApp which is configured to receive Diameter Requests from an external client.
The DiameterLuaService communicates with the DiameterApp using the DIAMETER-S-… messages.
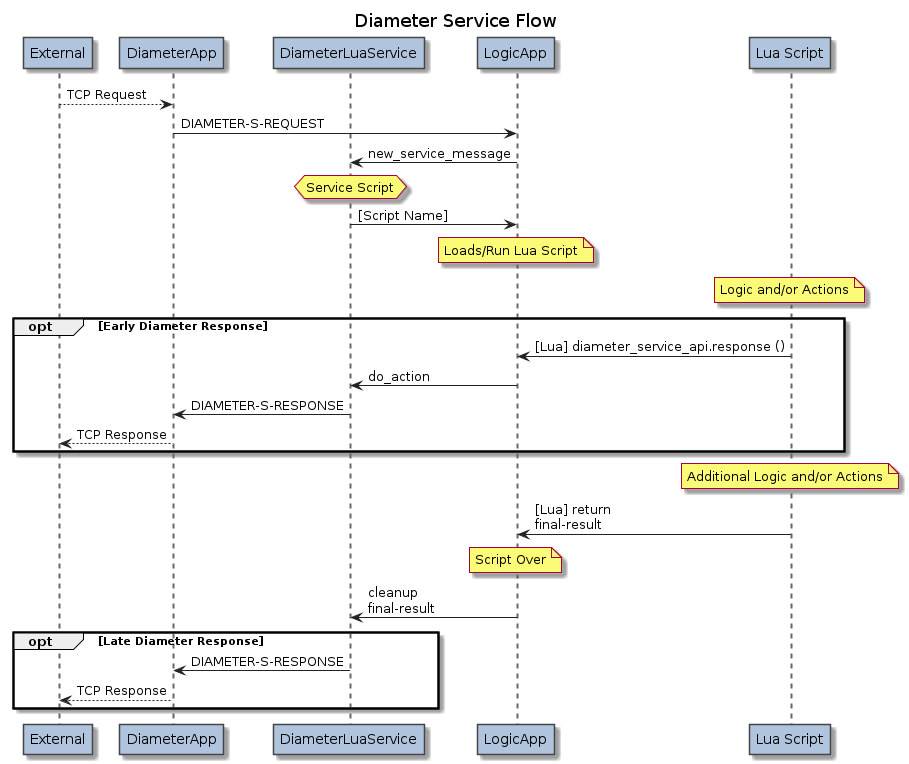
Configuring DiameterLuaService
The DiameterLuaService is configured within a LogicApp.
<?xml version="1.0" encoding="utf-8"?>
<n2svcd>
...
<applications>
...
<application name="Logic" module="LogicApp">
<include>
<lib>../apps/logic/lib</lib>
</include>
<parameters>
...
</parameters>
<config>
<services>
<service module="DiameterApp::DiameterLuaService" libs="../apps/diameter/lib" script_dir="/var/lib/n2svcd/logic/diameter"/>
</services>
<agents>
...
</agents>
</config>
</application>
...
</application>
...
</n2svcd>
In addition to the Common LogicApp Service Configuration, note the following specific attribute notes, and service-specific attributes.
Under normal installation, the following service
attributes apply:
Parmeter Name | Type | XML Type | Description |
---|---|---|---|
module
|
String | Attribute |
[Required] The module name containing the Lua Service code: DiameterApp::DiameterLuaService
|
libs
|
String | Element |
Location of the module for DiameterLuaService.(Default: ../apps/diameter/lib )
|
script_dir
|
String | Attribute | [Required] The directory containing scripts used by this service. |
Script Selection (Diameter Request)
Script selection is not configured. The script name is simply the Diameter message name received in the request.
e.g. an inbound request for message Credit-Control-Request
will map to a script name of Credit-Control-Request
,
which will be satisfied by a file named Credit-Control-Request.lua
in any of the directories
configured as lua_script_dir
in the LogicApp.
Refer to the LogicApp configuration for more information on directories, library paths, and script caching parameters.
Script Global Variables
Scripts run with this service have access to the Common LUA Service Global Variables.
There are no service-specific global variables.
Script Entry Parameters (Diameter Request)
The Lua script must be a Lua chunk such as the following:
local n2svcd = require "n2.n2svcd"
local diameter = ...
n2svcd.debug ("Echo Supplied Diameter Inputs:")
n2svcd.debug_var (diameter)
-- Echo back all of the received AVPs with Result-Code appended.
diameter.avps.insert ({ name = "Result-Code", value = 2001 })
return diameter.avps
The chunk will be executed with a single diameter
entry parameter which is an object with the
following attributes:
Field | Type | Description |
---|---|---|
.name
|
String |
The name of the Diameter command, e.g. Credit-Control if known.
|
.code
|
Integer | [Required] The numeric code for the command. |
.application_id
|
Integer | The received Application-ID from the request header. |
.avps
|
Array of Object | [Required] Array of AVP descriptors each with the following fields. |
[].name
|
String |
The name of Diameter AVP, e.g. Rating-Group , if known.
|
[].code
|
Integer | [Required] The code of the Diameter AVP. |
[].vendor_id
|
Integer | The vendor ID, if present. |
[].type
|
String |
[Required] One of None , OctetString , UTF8String , DiamIdent , Integer32 ,
Integer64 , Unsigned32 , Enumerated , Time , Unsigned64 ,
Address , Grouped .
|
[].mandatory
|
0 /1
|
Is the AVP specified as mandatory? |
[].encryption
|
0 /1
|
Is the AVP specified as encrypted? |
[].value
|
Various |
The value for this AVP. For AVPs of type Grouped this will be an ARRAY.For all other AVPs this is a SCALAR of the appropriate type. |
Script Return Parameters (Diameter Response)
The Lua script is responsible for determing the Diameter Response which will be sent back in reply to the original Diameter Request.
The simplest way to do this is by the return value given back to the service at the end of script execution.
The script return value should be an array of AVPs, where each array entry has the following attributes:
Field | Type | Description |
---|---|---|
[].name
|
String |
The name of Diameter AVP, e.g. Rating-Group .This must be a supported AVP name within N2::DIAMETER::Codec or DiameterApp custom AVPs.If your AVP name is not supported, you may instead specify [].code .
|
[].code
|
Integer |
The code of Diameter AVP, if not specifying by [].name .
|
[].vendor_id
|
Integer |
The vendor ID. This field is optional and can be specified both when
selecting the AVP by [].name (to distinguish between scenarios where the
same name is used by different vendors) or when selecting the AVP by [].code
in order to specify the intended vendor ID.
|
[].type
|
String |
One of None , OctetString , UTF8String , DiamIdent , Integer32 ,
Integer64 , Unsigned32 , Enumerated , Time , Unsigned64 ,
Address , Grouped .This is used only when specifying an AVP by [].code .For named AVPs the type is pre-defined. |
[].mandatory
|
0 /1
|
Override the default mandatory AVP flag. This is used only when specifying an AVP by [].code .For named AVPs the mandatory flag is pre-defined. |
[].encryption
|
0 /1
|
Override the default encryption AVP flag. This is used only when specifying an AVP by [].code .For named AVPs the encryption flag is pre-defined. |
[].value
|
Various |
The value for this AVP. For AVPs of type Grouped this will be an ARRAY.For all other AVPs this is a SCALAR of the appropriate type. |
Example (returning Diameter Response arguments):
local n2svcd = require "n2.n2svcd"
local diameter = ...
return {
{ name = "Custom-1", value = 789 },
{ name = "Result-Code", value = 2001 }
}
Note that returning nil
in this case would cause an empty Diameter response to be
returned, which is very unlikely to be a satisfactory behavior.
Note that the Diameter Lua script is responsible for adding the Result-Code
AVP,
along with all other AVPs.
The DiameterLuaService API
The Diameter Service API can be loaded as follows.
local diameter_service_api = require "n2.n2svcd.diameter_service"
It is not necessary to load the Diameter Service API if you are only using the simple response mechanism described above. It is only required if you wish to use any of the extended features described below.
.response
When a Lua Script needs to perform extended processing, it may wish to send an early Diameter
response before the script completes. This can be done with the response
method on the
Diameter Service API. This method also allows the script to use a non-default response message name.
The response
method takes the following parameters.
Parameter | Type | Description |
---|---|---|
avps
|
Array of Object | A Lua table with the same AVPs array structure as defined previously in the section describing the response mechanism. |
The response
method returns true
.
[Fragment] Example (Early Response with failure Result-Code):
...
diameter_service_api.response ({ name = "Result-Code", value = 5030 })
...
[post-processing after Diameter transaction is concluded]
...
return nil
A chunk should return nil
after an early response. The actual return result is
ignored in this scenario.